I am working on a solution that already has HTML sitemap as a part of Navigation feature. Now I got a request to add also a basic XML sitemap with common set requirements. Habitat ships with an interface template _Navigable
, so let's extend this template by adding a checkbox field called
ShowInSitemap
, stating whether a particular page will be shown in that sitemap:
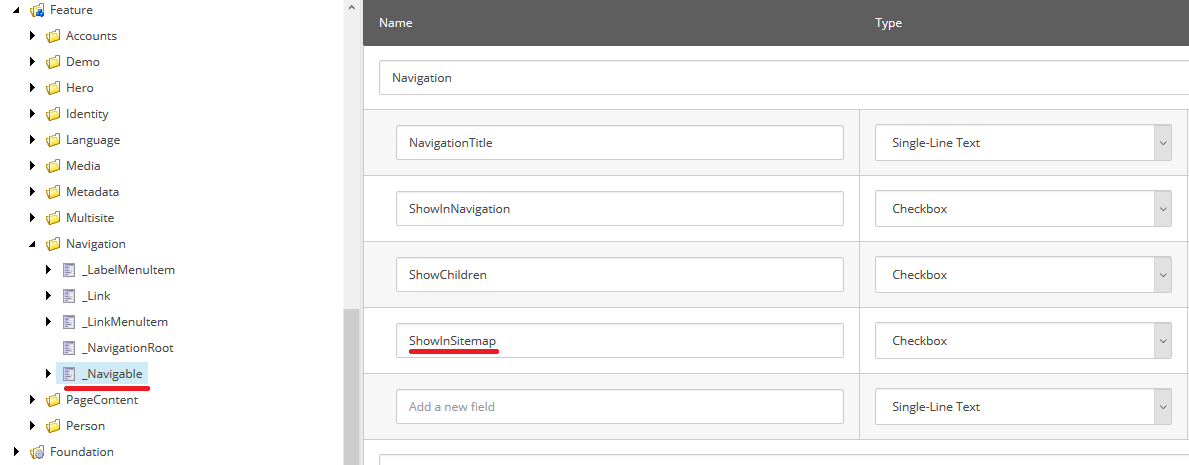
In order to start, we need to create a handler. Having handlers in web.config is not the desired way of doing things, it will require also doing configuration transform for the deployments, so let's do things in a Sitecore way (Feature.Navigation.config
file):
<configuration xmlns:patch="http://www.sitecore.net/xmlconfig/">
<sitecore>
<pipelines>
<httpRequestBegin>
<processor type="Platform.Feature.Navigation.Pipelines.SitemapHandler, Platform.Feature.Navigation"
patch:before="processor[@type='Sitecore.Pipelines.HttpRequest.CustomHandlers, Sitecore.Kernel']">
</processor>
</httpRequestBegin>
<preprocessRequest>
<processor type="Sitecore.Pipelines.PreprocessRequest.FilterUrlExtensions, Sitecore.Kernel">
<param desc="Allowed extensions">aspx, ashx, asmx, xml</param>
</processor>
</preprocessRequest>
</pipelines>
</sitecore>
</configuration>
We rely on httpRequestBegin
pipeline and incline our new SitemapHandler from Navigation feature right before CustomHandlers processor.
SitemapHandler is an ordinary pipeline processor for httpRequestBegin pipeline, so is inherited from HttpRequestProcessor:
public class SitemapHandler : HttpRequestProcessor
{
const string sitemapHandler = "sitemap.xml";
private readonly INavigationRepository _navigationRepository;
public SitemapHandler()
{
_navigationRepository = new NavigationRepository(RootItem);
}
public override void Process(HttpRequestArgs args)
{
if (Context.Site == null
|| args == null
|| string.IsNullOrEmpty(Context.Site.RootPath.Trim())
|| Context.Page.FilePath.Length > 0
|| !args.Url.FilePath.Contains(sitemapHandler))
{
return;
}
Response.ClearHeaders();
Response.ClearContent();
Response.ContentType = "text/xml";
try
{
var navigationItems = _navigationRepository.GetSitemapItems(RootItem);
string xml = new XmlSitemapService().BuildSitemapXML(flatItems);
Response.Write(xml);
}
finally
{
Response.Flush();
Response.End();
}
}
private Item RootItem => Context.Site.GetRootItem();
private HttpResponse Response => HttpContext.Current.Response;
}
And XmlSitemapService
code below:
public class XmlSitemapService
{
public string CreateSitemapXml(IEnumerable<NavigationItem> items)
{
var doc = new XmlDocument();
var declarationNode = doc.CreateXmlDeclaration("1.0", "UTF-8", null);
doc.AppendChild(declarationNode);
var urlsetNode = doc.CreateElement("urlset");
var xmlnsAttr = doc.CreateAttribute("xmlns");
xmlnsAttr.Value = "http://www.sitemaps.org/schemas/sitemap/0.9";
urlsetNode.Attributes.Append(xmlnsAttr);
doc.AppendChild(urlsetNode);
foreach (NavigationItem itm in items)
{
doc = CreateSitemapRecord(doc, itm);
}
return doc.OuterXml;
}
private XmlDocument CreateSitemapRecord(XmlDocument doc, NavigationItem item)
{
string link = item.Url;
string lastModified = HttpUtility
.HtmlEncode(item.Item.Statistics.Updated.ToString("yyyy-MM-ddTHH:mm:sszzz"));
XmlNode urlsetNode = doc.LastChild;
XmlNode url = doc.CreateElement("url");
urlsetNode.AppendChild(url);
XmlNode loc = doc.CreateElement("loc");
url.AppendChild(loc);
loc.AppendChild(doc.CreateTextNode(link));
XmlNode lastmod = doc.CreateElement("lastmod");
url.AppendChild(lastmod);
lastmod.AppendChild(doc.CreateTextNode(lastModified));
return doc;
}
}
Also, NavigationItem
is a custom POCO:
public class NavigationItem
{
public Item Item { get; set; }
public string Title { get; set; }
public string Url { get; set; }
public bool IsActive { get; set; }
public int Level { get; set; }
public NavigationItems Children { get; set; }
public string Target { get; set; }
public bool ShowChildren { get; set; }
}
Few things to mention.
1. Since you are using LinkManager in order to generate the links, you need to make sure you have full URL path as required by protocol, not the site-root-relative path. So you'll need to pass custom options in that case:
2. Once deployed to production, you may face an unpleasant behavior of HTTPS links generated along with 443 port number (such as . That is thanks to LinkManager not being wise enough to predict such a case. However there is a setting that make LinkManager works as expected. Not obvious
var options = LinkManager.GetDefaultUrlOptions();
options.AlwaysIncludeServerUrl = true;
options.SiteResolving = true;
LinkManager.GetItemUrl(item, options);
or better option in Heliix to rely on Sitecore.Foundation.SitecoreExtensions:
item.Url(options) from
//TODO: Update the code with the recent
That's it!
When creating websites with Sitecore it is always nice to a have a nice and shiny 404-page that complies with the website styles and is content editable. Which will not work in all the cases, unfortunately. If the request falls out of site context - user won't get that nice and shiny page. So let's see what we can do in Helix, as it assumes multisite by design. The code below is written on top of Habitat, but that should not make a big difference.
Since this functionality is website agnostic and relates to Project layer, we will be using Project.Common.Website. Here is the configuration within Common.Website.config
:
<httpRequestBegin>
<processor patch:before="processor[@type='Sitecore.Pipelines.HttpRequest.LayoutResolver, Sitecore.Kernel']"
type="Sitecore.Common.Website.Pipelines.HandleRequestError, Sitecore.Common.Website"
patch:after="processor[@type='Sitecore.Pipelines.HttpRequest.ItemResolver, Sitecore.Kernel']" />
</httpRequestBegin>
And the processor HandleRequestError.cs
:
public class HandleRequestError : HttpRequestProcessor
{
public override void Process(HttpRequestArgs args)
{
if (Context.Item != null || Context.Site == null)
{
return;
}
string filePath = HttpContext.Current.Server.MapPath(args.Url.FilePath);
if (IsValidItem()
|| ReservedUrls.Includes(args.Url.FilePath)
|| File.Exists(filePath))
{
return;
}
if (Context.Database != null)
{
// if we are in a context of a specific site - serve content-editable 404
Context.Item = Context.Database.GetItem(Context.Site.StartPath + "/404");
}
// return 404 HTTP status code in either cases, to be picked up further ahead
HttpContext.Current.Response.TrySkipIisCustomErrors = true;
HttpContext.Current.Response.StatusCode = (int)HttpStatusCode.NotFound;
}
private bool IsValidItem()
{
if (Context.Item == null || Context.Item.Versions.Count == 0) return false;
if (Context.Item.Visualization.Layout == null) return false;
return true;
}
}
So briefly, if we have website resolved - we serve its customized content-editable /404 page item (each of the sites has this page under the same root-relative path).
Recently tried to reinstall Sitecore 9.0 update 1 and got the following message:
"Request failed: Unable to connect to remote server" (and the URL which is default https://localhost:8389/solr)
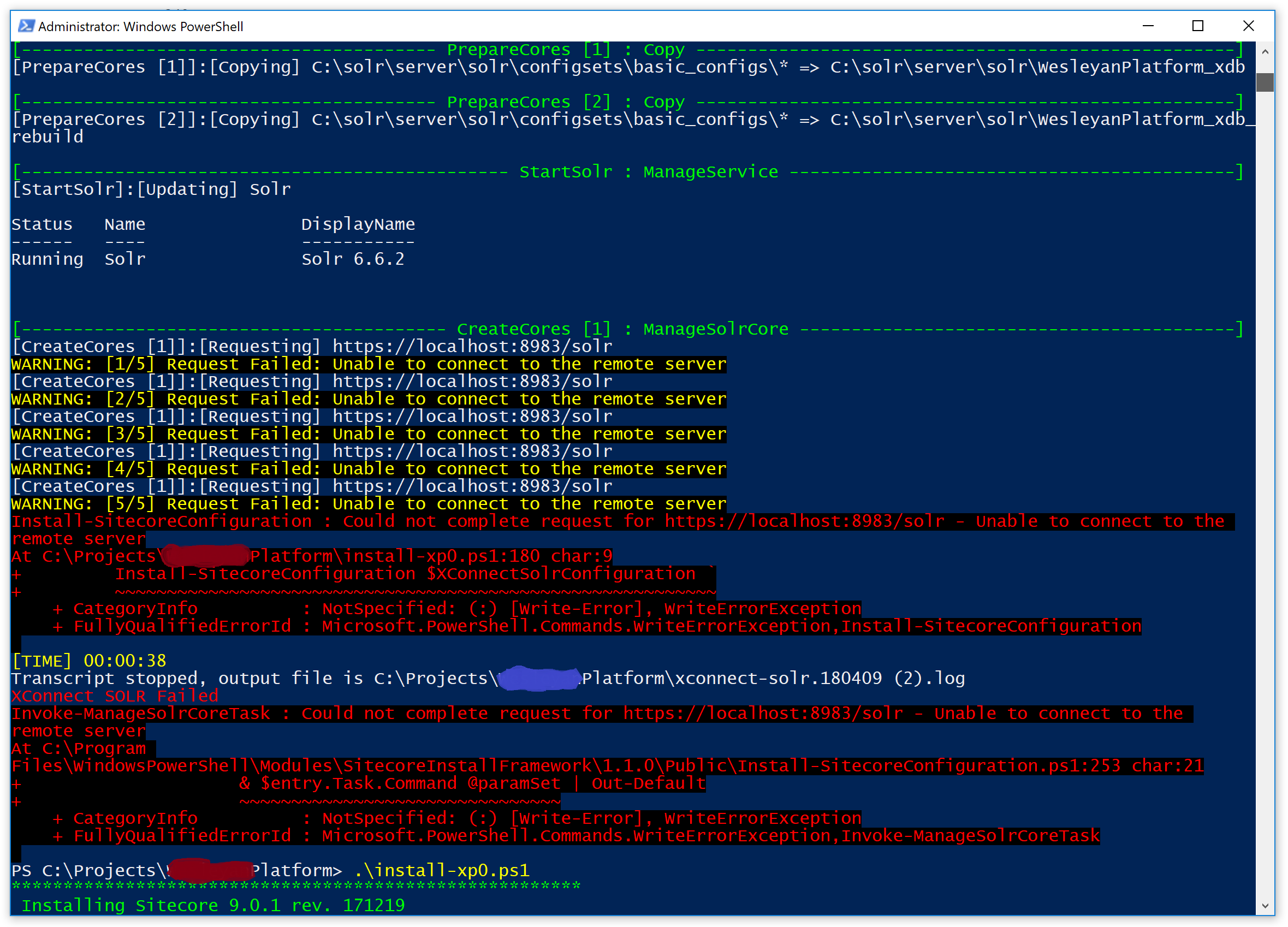
Opening Solr in browser went well, and there were no existing cores that could prevent the installation. Weird...
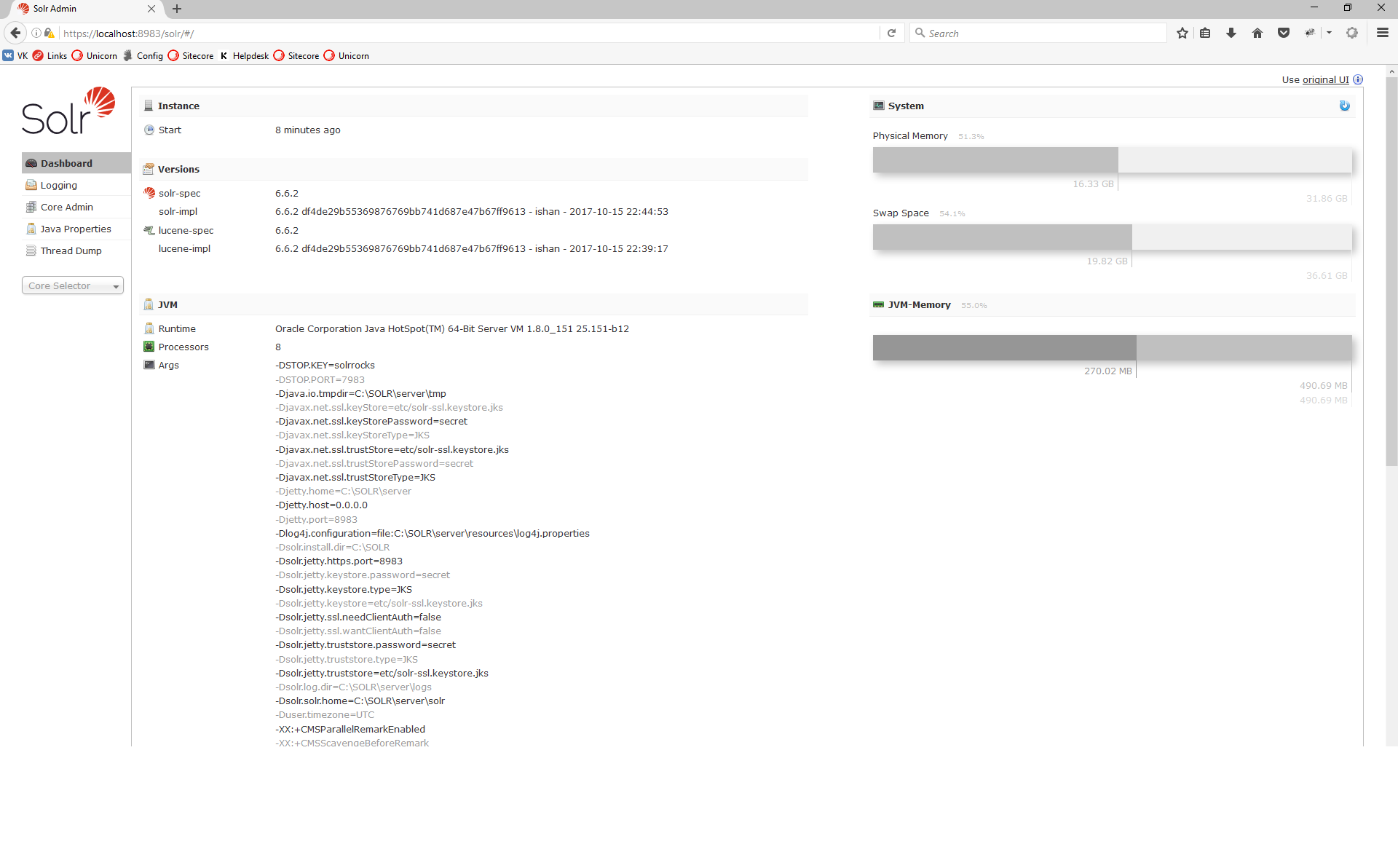
After experiments, I found out that was an antivirus preventing such requests. Disabling it allowed cores to be installed well and thу rest of install script succeeded.