Recently I have inherited a project that utilised dynamic placeholder in a weird way:
public static HtmlString DynamicPlaceholder(this SitecoreHelper helper, string placeholderKey)
{
if (string.IsNullOrEmpty(RenderingContext.Current.Rendering?.DataSource))
return helper.Placeholder(placeholderKey);
var currentRenderingId = Guid.Parse(RenderingContext.Current.Rendering.DataSource);
return helper.Placeholder($"{placeholderKey}_{currentRenderingId}");
}
instead of more commonly used way suggested by Jason Bert:
namespace DynamicPlaceholders.Mvc.Extensions
{
public static class SitecoreHelperExtensions
{
public static HtmlString DynamicPlaceholder(this SitecoreHelper helper, string placeholderName)
{
string text = PlaceholdersContext.Add(placeholderName, RenderingContext.Current.Rendering.UniqueId);
return helper.Placeholder(text);
}
}
}
In two words, the first approach generates placeholder name using an ID of datasource item. The second approach is more traditional and is default for Helix. It was suggested by Jason Bert and also utilised by sitecore - that relies on rendering's UniqueID. In both cases, dynamic placeholders look like: /content/name-of-placeholder_017c3643-0fef-475c-95d2-bb1107beb664.
So I need to update it across entire solution. Let's iterate our items.
First of all, I do not need to go through all of item, but only those that are pages and have presentation configured, as I am going to adjust it. Secondly, those items are located under /sitecore/content folder. As many of you should know, presentation details (or deltas) for a page are kept within two fields of Standard Template - Renderings and Final Renderings that correspond to configuration you see at Shared Layout and Final Layout tabs. These thoughts resulted in using following Sitecore query to identify those items:
/sitecore/content//*[@__Renderings != '' or @__Final Renderings != '']
So far, so good. Also need to mention that I will perform the operation for master database only and for Default device:
private const string DatabaseName = "master";
private const string DefaultDeviceId = "{FE5D7FDF-89C0-4D99-9AA3-B5FBD009C9F3}";
I am using Sitecore presentation API in order to achieve my goal. Below is my Iterate() method:
public Dictionary<Item, List<KeyValuePair<string, string>>> Iterate()
{
var result = new Dictionary<Item, List<KeyValuePair<string, string>>>();
var master = Factory.GetDatabase(DatabaseName);
var items = master.SelectItems(ItemsWithPresentationDetailsQuery);
var layoutFields = new[] {FieldIDs.LayoutField, FieldIDs.FinalLayoutField};
foreach (var item in items)
{
foreach (var layoutField in layoutFields)
{
var changeResult = ChangeLayoutFieldForItem(item, item.Fields[layoutField]);
if (changeResult.Any())
{
if (!result.ContainsKey(item))
{
result.Add(item, changeResult);
}
else
{
result[item].AddRange(changeResult);
}
}
}
}
return result;
}
That method iterates through each item from returned from the query and calls ChangeLayoutFieldForItem for that item twice - for both presentation fields Renderings and Final Renderings.
private List<KeyValuePair<string, string>> ChangeLayoutFieldForItem(Item currentItem, Field field)
{
var result = new List<KeyValuePair<string, string>>();
string xml = LayoutField.GetFieldValue(field);
if (!string.IsNullOrWhiteSpace(xml))
{
LayoutDefinition details = LayoutDefinition.Parse(xml);
var device = details.GetDevice(DefaultDeviceId);
DeviceItem deviceItem = currentItem.Database.Resources.Devices["Default"];
RenderingReference[] renderings = currentItem.Visualization.GetRenderings(deviceItem, false);
var datasourceGuidsToRenderingUniqueIdMap = renderings
.Where(r => !string.IsNullOrWhiteSpace(r.Settings.DataSource))
.Select(r => new KeyValuePair<string, string>(Guid.Parse(r.Settings.DataSource).ToString(), r.UniqueId));
if (device?.Renderings != null)
{
foreach (RenderingDefinition rendering in device.Renderings)
{
if (!string.IsNullOrWhiteSpace(rendering.Placeholder))
{
var verifiedPlaceholderKey = FixPlaceholderKey(rendering.Placeholder, datasourceGuidsToRenderingUniqueIdMap);
result.Add(new KeyValuePair<string, string>(rendering.Placeholder, verifiedPlaceholderKey));
rendering.Placeholder = verifiedPlaceholderKey;
}
}
string newXml = details.ToXml();
using (new EditContext(currentItem))
{
LayoutField.SetFieldValue(field, newXml);
}
}
}
return result;
}
Further down it creates a list of matches for all the renderings within current presentation field of that particular item - datasource GUIDs matching to unique rendering IDs to go through it and do a replacement - that is done in FixPlaceholderKey method. Once everything is replaced, save the field by calling LayoutField.SetFieldValue() of course wrapping that call with EditContext as we are modifying item's field value.
FixPlaceholderKey is a simple method that just does case insensitive replacement by using Regex:
private string FixPlaceholderKey(string renderingInstancePlaceholder, IEnumerable<KeyValuePair<string, string>> map)
{
var value = renderingInstancePlaceholder;
foreach (var oldValue in map)
{
value = Regex.Replace(value, oldValue.Key, Guid.Parse(oldValue.Value).ToString(), RegexOptions.IgnoreCase);
}
return value;
}
That is pretty everything about the dynamic placeholder fixing logic.
But as for my case - I was aware that I'll need to run this dynamic placeholder replacements few more times in future and will likely need to implement some other similar small tools. So I decided that it would be great to place it under /sitecore/admin folder along with other admin tools - exact location by purpose! And since we're now going Helix, I decided to follow good principles and created a foundation module called AdminTools, where I will be adding similar admin folder tools. So here's how it looks for me in the Solution Explorer:
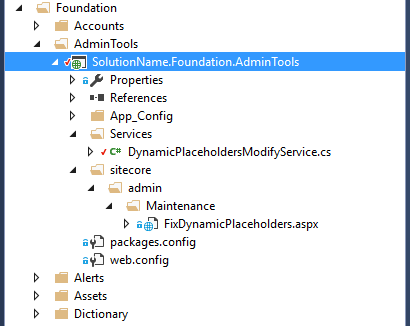
FixDynamicPlaceholders.aspx is a classical ASP.NET WebForm with one line markup (ah-h, I was so lucky not to deal with Webforms for couple past years till the moment)
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="FixDynamicPlaceholders.aspx.cs" Inherits="HomeServeUsa.Foundation.AdminTools.sitecore.admin.Maintenance.FixDynamicPlaceholders" %>
and the codebehind. Since FixDynamicPlaceholders.aspx is admin tool page - codebehind is inherited from Sitecore.sitecore.admin.AdminPage:
public partial class FixDynamicPlaceholders : AdminPage
{
protected void Page_Load(object sender, EventArgs e)
{
var fixRenderings = new DynamicPlaceholdersModifyService();
var result = fixRenderings.Iterate();
OutputResult(result);
}
private void OutputResult(Dictionary<Item, List<KeyValuePair<string, string>>> result)
{
Response.ContentType = "text/html";
Response.Write($"<h1>{result.Count} items processed</h1>");
foreach (var pair in result)
{
Response.Write($"<h3>{pair.Key.Paths.FullPath}</h3>");
foreach (var kvp in pair.Value)
{
if (kvp.Key != kvp.Value)
{
Response.Write($"<div>{kvp.Key} ==> {kvp.Value}</div>");
}
}
}
}
}
Finally, it is complete. Hope this helps someone!
P.S. of course, that would be easier (and more elegant) to perform with Sitecore PowerShell extension. But unfortunately I am the only person in the organisation who uses it regardless of my promotional activity.