In previous post we have created an MVC form, that submits to a feature controller and set up the routing to make it all work. In this part we'll add validation to that form. This part does not differ from traditional MVC approach, however let's make our form smooth and complete.
1. Add validation controls into a page for each of inputs:
@using (Html.BeginRouteForm(MvcSettings.SitecoreRouteName, FormMethod.Post))
{
@Html.LabelFor(x => x.FirstName)
@Html.TextBoxFor(x => x.FirstName)
@Html.ValidationMessageFor(x => x.FirstName)
@Html.LabelFor(x => x.LastName)
@Html.TextBoxFor(x => x.LastName)
@Html.ValidationMessageFor(x => x.LastName)
@Html.LabelFor(x => x.Email)
@Html.TextBoxFor(x => x.Email)
@Html.ValidationMessageFor(x => x.Email)
}
2. Model needs to be updated with validation action filter attributes, provided by DataAnnotations:
using System.ComponentModel.DataAnnotations;
namespace YourSolution.Feature.Test.Models
{
public class TestModel
{
[Display(Name = nameof(FirstNameLabel), ResourceType = typeof(TestModel))]
[Required(ErrorMessageResourceName = nameof(Required), ErrorMessageResourceType = typeof(TestModel))]
[MinLength(3, ErrorMessageResourceName = nameof(MinimumLength), ErrorMessageResourceType = typeof(TestModel))]
public string FirstName { get; set; }
[Display(Name = nameof(LastNameLabel), ResourceType = typeof(TestModel))]
[Required(ErrorMessageResourceName = nameof(Required), ErrorMessageResourceType = typeof(TestModel))]
[MinLength(3, ErrorMessageResourceName = nameof(MinimumLength), ErrorMessageResourceType = typeof(TestModel))]
public string LastName { get; set; }
[Display(Name = nameof(EmailLabel), ResourceType = typeof(TestModel))]
[EmailAddress(ErrorMessageResourceName = nameof(InvalidEmailAddress), ErrorMessageResourceType = typeof(TestModel))]
[Required(ErrorMessageResourceName = nameof(Required), ErrorMessageResourceType = typeof(TestModel))]
public string Email { get; set; }
// Labels and validation messages: instead of hardcoded you may take it from Dictionary
public static string FirstNameLabel => "First name";
public static string LastNameLabel => "Last name";
public static string EmailLabel => "E-mail";
public static string Required => "Please enter a value";
public static string MinimumLength => "Should be at east 3 characters";
public static string InvalidEmailAddress => "please enter valid email address";
}
}
3. Last but not the least, need to decorate POST action with validation action filter attribute, that runs validation prior to executing controller and returns validated view model on failure or runs into controller action if there no errors. So, updated controller action:
[HttpPost]
[ValidateModel]
public ActionResult Test(TestModel loginInfo)
{
// do something on successful form submission
return new RedirectResult("/SuccessfulResultPage");
}
4. And of course, the ValidateModelAttribute
class itself:
using System.Web.Mvc;
namespace YourSolution.Feature.Test.Attributes
{
public class ValidateModelAttribute : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
var viewData = filterContext.Controller.ViewData;
if (!viewData.ModelState.IsValid)
{
filterContext.Result = new ViewResult
{
ViewData = viewData,
TempData = filterContext.Controller.TempData
};
}
}
}
}
5. After running gulp commands for updating views and DLL libraries, an updated page would do validation similar to:
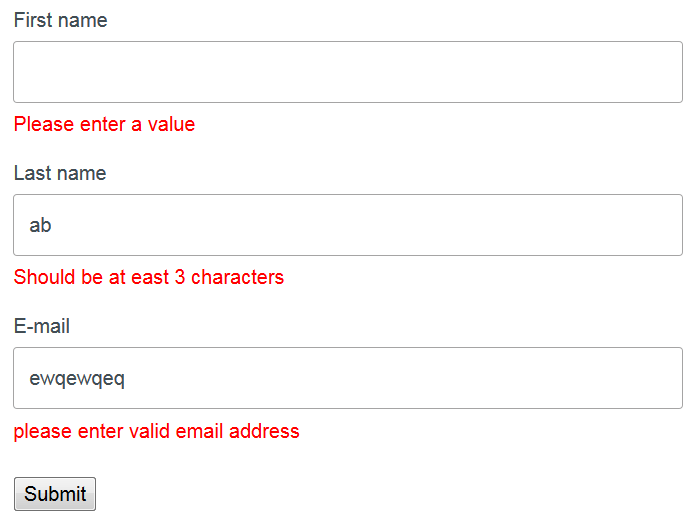
That's it!
Finally, our feature project named Test would have the following structure in Solution Explorer:
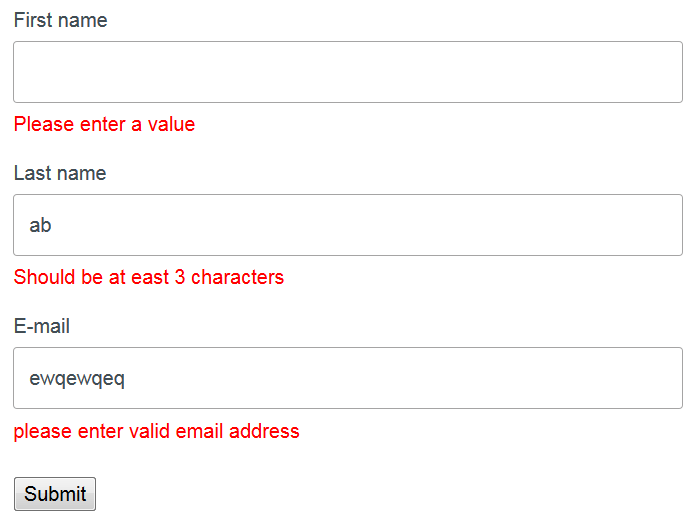
Thanks for reading!